SSL Security Analysis
In today's cybersecurity landscape, ensuring SSL certificate integrity and domain security is crucial for businesses, website owners, and IT security teams. Manually analyzing SSL certificates and identifying vulnerabilities can be time-consuming and prone to oversight.
Traditional Approach Challengesβ
SSL security audits are typically conducted manually by security teams or system administrators. These traditional methods come with notable challenges:
- Certificate Enumeration: Security professionals must manually query SSL certificate databases like crt.sh to retrieve certificate details for a given domain.
- Risk Analysis: Identifying potential vulnerabilities in SSL configurations requires expertise and manual review of certificate issuers, expiration dates, and security policies.
- Report Generation: Creating structured reports with risk assessments, compliance checks, and security recommendations is a time-intensive task.
Note: This use case automates SSL certificate retrieval, analysis, and report generation while leaving final security decisions to human experts.
The Agentic Solutionβ
KaibanJS automates SSL security analysis by leveraging intelligent agents that fetch and analyze SSL certificates, generate security reports, and recommend improvements.
- Scenario: Auditing SSL certificates for a given domain to ensure compliance, security, and proper configuration.
Before diving into the automated process, letβs introduce the key Agent responsible for this task:
- SSL Certificate Analyzer:
- Retrieves certificates using crt.sh.
- Analyzes certificate details, including issuers, validity periods, and risks.
- Identifies subdomains associated with the target domain.
- Generates a structured security report with recommendations.
Process Overviewβ
Hereβs how the agent-driven workflow automates SSL certificate analysis:
- Certificate Retrieval: The agent queries crt.sh via a proxy to fetch SSL certificate data for the target domain.
- Security Analysis: Extracts subdomain information, certificate issuers, expiration dates, and potential misconfigurations.
- Risk Assessment: Flags vulnerabilities such as outdated certificates, wildcard usage risks, and compatibility issues.
- Report Generation: Creates a structured SSL security report in markdown format, highlighting risks and recommended actions.
Implementationβ
Below is an example of how this automation is implemented using KaibanJS:
import { Agent, Task, Team } from 'kaibanjs';
import { Tool } from "@langchain/core/tools";
import { z } from "zod";
import axios from "axios";
// === CRTTool ===
export class CRTTool extends Tool {
constructor(fields) {
super(fields);
this.name = "crt_tool";
this.description = "Fetches JSON data of certificates from crt.sh for a given domain using a CORS proxy.";
this.schema = z.object({
domain: z
.string()
.describe("The domain to fetch certificate JSON data for."),
});
}
async _call(input) {
try {
const requestUrl = `https://api.allorigins.win/raw?url=${encodeURIComponent(
`https://crt.sh/json?q=${input.domain}`
)}`;
const response = await axios.get(requestUrl);
// Parse the raw JSON response
const data = response.data;
if (typeof data === 'string') {
return JSON.parse(data); // Ensure string JSON is parsed
}
return data;
} catch (error) {
return `Error fetching JSON data from crt.sh: ${error.message}`;
}
}
}
// Tool instance
const crtTool = new CRTTool();
// === AGENTS ===
const sslAnalyzer = new Agent({
name: 'SSL Certificate Analyzer',
role: 'SSL Certificate Security Analyst',
goal: 'Analyze SSL certificates and generate detailed security reports',
background: 'Expert in SSL certificate analysis and domain security configuration',
description: `Analyzes SSL certificate data issued for the domain {domain} and generates a report in {language}. Provides a report including:
- List of identified subdomains
- Information about certificate issuers and validity periods
- Potential risks related to subdomains and SSL configuration
- Recommendations to improve domain security`,
tools: [crtTool],
});
// === TASKS ===
const analyzeSSL = new Task({
name: 'analyze_ssl',
description: 'Analyzes SSL certificates for {domain} and generates a security report in {language}',
agent: sslAnalyzer,
expectedOutput: `Report template:
[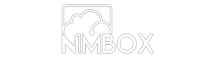](https://nimbox360.com)
# π SSL Security Report for {domain}
*Generated by [Nimbox360](https://nimbox360.com) with KaibanJS*
## π Executive Summary
[Brief description of analysis and key findings]
## π Identified Subdomains
- List of found subdomains
- Each on a separate line with bullet point
## π SSL Certificates
| Domain | Issuer | Valid From | Valid Until | Status |
|---------|---------|--------------|--------------|---------|
[Table with certificate information]
### β οΈ Certificate Compatibility
If Let's Encrypt certificates are detected:
- β Let's Encrypt certificates are not compatible with older iPhone devices
- β May present issues with older operating system versions
- π‘ Consider commercial certificates for broader compatibility
## π¨ Risk Analysis
1. [Identified Risk]
- Risk details
- Potential impact
2. [Next risk...]
### π Information Exposure
- Evaluation of critical subdomain exposure
- Identification of potential entry points for attackers
## π‘ Recommendations
1. **Wildcard Certificates**
- π‘οΈ Use wildcard certificates (*.domain.com) to hide specific subdomains
- π Reduces exposure of sensitive information
- π Simplifies certificate management
2. **Security Improvements**
- π Implement automatic certificate renewal
- π‘οΈ Configure HSTS to prevent attacks
- π Keep SSL/TLS configurations up to date
## π Conclusions
[Summary of findings and recommended next steps]
---
*π Analysis performed by [Nimbox360](https://nimbox360.com) SSL Analyzer*`
});
// === TEAM ===
const team = new Team({
name: 'SSL Security Team',
agents: [sslAnalyzer],
tasks: [analyzeSSL],
inputs: {
domain: 'kaibanjs.com', // Domain to analyze SSL certificates
language: 'english', // Language for the report (english, spanish)
},
env: {
OPENAI_API_KEY: import.meta.env.VITE_OPENAI_API_KEY ?? (() => { throw new Error('OPENAI_API_KEY is required') })(),
}
});
export default team;
Outcomeβ
The result is an automatically generated SSL Security Report that provides:
- A list of subdomains associated with the target domain.
- Certificate issuer details, validity periods, and expiration warnings.
- Identification of potential security risks and misconfigurations.
- Actionable recommendations for improving SSL security posture.
By leveraging KaibanJS for SSL security analysis, cybersecurity teams and IT administrators can streamline their workflow, reduce manual effort, and improve security monitoring with AI-powered automation.
Expected Benefitsβ
- Automated Security Audits: Reduce manual effort by automating SSL certificate retrieval and analysis.
- Faster Threat Detection: Quickly identify security risks related to SSL misconfigurations.
- Structured Compliance Reporting: Generate consistent and detailed security reports for audit and compliance purposes.
- Enhanced Domain Protection: Improve overall web security by proactively identifying vulnerabilities.
Get Started Todayβ
Ready to enhance your SSL security assessment workflow? Start using KaibanJS today and explore its powerful AI-driven capabilities.
π Website: KaibanJS
π» GitHub Repository: KaibanJS on GitHub
π€ Discord Community: Join the Community
Help improve this use case by submitting an issue on GitHub. We appreciate your input!